grunt-nyc-mocha
grunt-nyc-mocha uses the third-party tools nyc and mocha, to setup a combined run of tests and coverage analysis.
It will stop a build process on failed tests as well as on unreached coverage goals.
The below shows the result of a successful run.
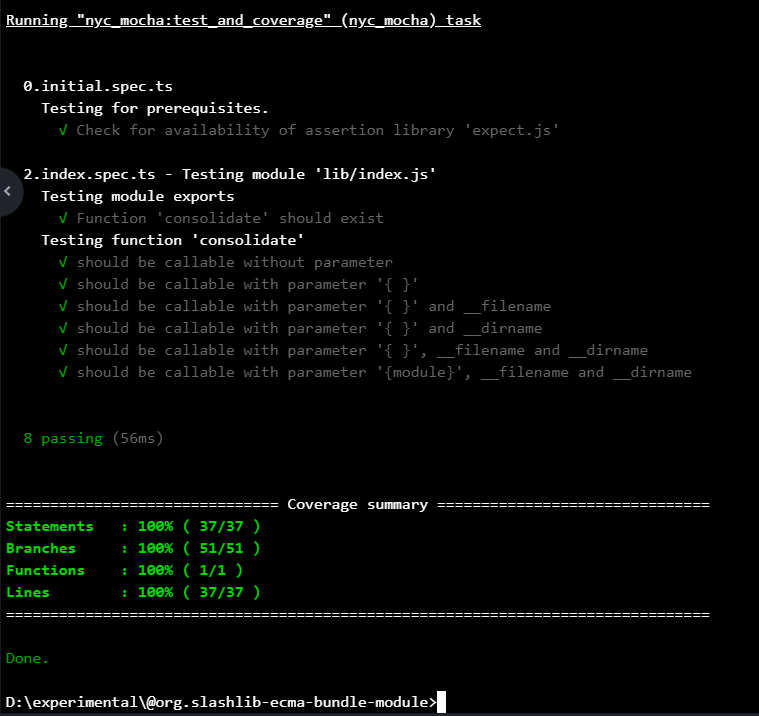
Coverage results can additionally be reported in multiple formats. HTML for example.
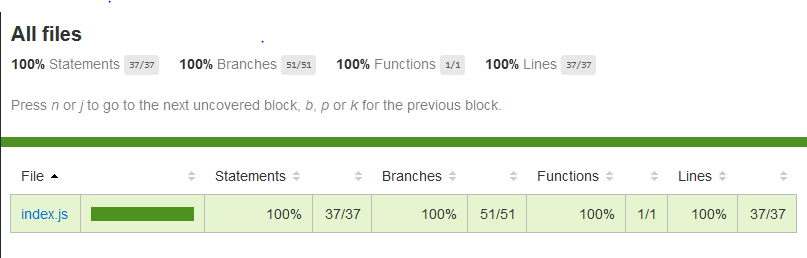
grunt-nyc-mocha provides full sourcemap support.
grunt-nyc-mocha takes on the role of calling the packages nyc and mocha, passing configuration parameters from grunt to them.
grunt-nyc-mocha is open source software (OSS) and distributed under the MIT License.
Third party tools:
nyc is open source software (OSS) and distributed under the ISC License.
mocha is open source software (OSS) and distributed under the MIT License.
Prerequisites for installing grunt-nyc-mocha are an existing npm that ships with nodejs and grunt.
npm install grunt-nyc-mocha --save-dev
Configuration of the plugin is usually done via an entry in the grunfile.js file. To be able to keep an overview of the configuration even when using many plugins, we use the package load-grunt-config. This way we can keep a separate configuration file for each plugin, as the following example shows.
// file nyc_mocha.js module.exports = function ( grunt, options ) { return { test_and_coverage: { src: `${ options.TESTDIR }/**/*.spec.js`, // test suite to run... options: { nyc: { coverage: { // report nyc coverage results dir: `${ options.COVERAGEDIR }`, // ... to folder reporter: [ "lcov", "cobertura", "text-summary" ], // ... using reporters check: true, perfile: true, branches: 100, functions: 100, lines: 100, statements: 100 }, excludes: [ "gruntfile.js", ".conf/**/*.js", "src/test/**/*.js" ], requires: [ "grunt-nyc-mocha/scripts/sourcemapsupport" ] }, mocha: { color: true // force colored output } } } }; };
grunt-nyc-mocha
Which parameters can be passed from grunt-nyc-mocha to nyc and mocha?
All!
The configuration section nyc contains a property
, which can be passed an array of strings. All strings in the array will be passed to nyc if the task is called.opts
The configuration section mocha contains a property
, which can be passed an array of strings. All strings in the array will be passed to mocha if the task is called.opts
If grunt-nyc-mocha itself might be outdated one day, any parameter can be passed to nyc and mocha. Even those not known to the outdated plugin.
Currently the following parameters of nyc are supported:
–branches
–cwd
–check-coverage
–clean
–exclude
–extension
–functions
–include
–lines
–per-file
–produce-source-map
–reporter
–report-dir
–require
–source-map
–statements
–temp-dir
As well as the following parameters of mocha:
–bail
–color
–exit
–recursive
–timeout
–ui
Property <targetname> {Object}
grunt-check-outdated is a multitask plugin, which means, there can be any number of targets to run.
Each target requires a number of properties.
// file nyc_mocha.js module.exports = function ( grunt, options ) { return { test_and_coverage: { // ... further properties go here! } }; };
grunt
Because grunt tasks need to be named,
is the name of the only task configured in this example. You could also use foo, bar or another string instead.test_and_coverage
Property src {string}
The src property is of type string and holds a path or glob, which defines the tests to be run by grunt-nyc-mocha.
Infact mocha will be used to run and report the tests, while nyc will takeover the part of instrumenting the sourcefiles and report coverage.
// file nyc_mocha.js module.exports = function ( grunt, options ) { return { test_and_coverage: { src: `${ options.TESTDIR }/**/*.spec.js`, // test suite to run... options: { <...> // see property options for more } } }; };
grunt-nyc-mocha
All coverage data is collected by running tests, during which library sources will be called.
This is, why targets must specify the tests to run.
Testfiles require sourcefiles, which then will be instrumented by nyc.
Code instrumentation enables nyc to build code metrics and coverage reports
Without the help of sourcemaps, failing tests will raise exceptions pointing to instrumented code, which differs from the original sourcefiles.
Resulting errormessages and stacktraces are hard to interpret, which is why you should use sourcemap support.
(See option
for more)nyc.requires
Property options {Object}
This property can be configured for all tasks or per task.
The example sets options for the task test_and_coverage
.
// file nyc_mocha.js module.exports = function ( grunt, options ) { return { test_and_coverage: { options: { cwd: {false|string} // current working directory dryrun: {Boolean:false} // show what you would do, if you should node: { // see property node for more options: {Array<string>}, // array of commandline options for node exec: {string} // path to node.exe }, nyc: { <...> // see property nyc for more }, mocha: { <...> // see property mocha for more }, } } }; };
grunt
Options for running grunt-nyc-mocha can have three sections.
Each section is for configuring the named third-party-tool.
Property
(current working directory)cwd {path}
grunt-nyc-mocha needs to know the directory where the package.json
file is located. If grunt is started in another directory, the cwd property must be set.
Usually the value can be evaluated and fits automatically, meaning it does not need to be set manually.
Property dryrun {Boolean}
If the value of the property is set to true, check-outdated is no longer called. Instead, the string that would have started the third-party tools will be written to the console.
The output should be directly callable from the project’s commandshell.
Property node {Object}
The third-party tools are called in a separate node process. If it is necessary to make changes to the type of call, this can be done via the properties of the node object.
grunt-check-outdated
These are the standard features offered by each of our plugins.
They are independent of the third-party tools, but refer to the way they will be called.
Property nyc {Object}
The nyc property is of type object and bundles all properties, which later will be passed on to the third-party-tool nyc.
// nyc section const nyc = { all: <...>, // whether or not to instrument all files clean: <...>, // clean .nyc_output folder before testing coverage: { // coverage options <...> // see property coverage for details }, excludes: [ ... ], // array of files and directories to exclude exec: <...>, // path to node_modules/.../nyc script extensions: [ ... ], // additional extensions that nyc should handle includes: [ ... ], // array of files and directories to include opts: [ ... ], // additional options not covered by this grunt plugin requires: [ ... ], // array of scripts to additionally require sourcemap: { // sourcemap options <...> // see property sourcemap for more } temp: <...> // directory to output raw coverage information };
grunt-nyc-mocha
Which parameters can be passed from grunt-nyc-mocha to nyc?
All!
The configuration section nyc contains a property
, which can be passed an array of strings. All strings in the array will be passed to nyc if the task is called.opts
Property all {Boolean:false}
Whether or not nyc should instrument all files (not just the ones touched by the test suite).
// nyc section const nyc = { all: <...>, // whether or not to instrument all files };
nyc
Property clean {Boolean:false}
nyc sets up a cache folder. If you did not use grunt-contrib-clean earlier, as part of a build process for removing cache folders, you can choose to delete the folder by setting the option to true.
// nyc section const nyc = { clean: <...>, // clean .nyc_output folder before testing };
nyc
Property coverage {Object}
The coverage object bundles all properties which influence code coverage handling.
// nyc section const coverage = { branches: {Number:integer}, // % of branches expected to be covered? check: {Boolean}, // check wether coverage is within thresholds dir: {String:path}, // report nyc coverage results to folder functions: {Number:integer}, // % of functions expected to be covered? lines: {Number:integer}, // % of lines expected to be covered? perfile: {Boolean}, // check thresholds per file reporter: {Array<string>}, // report coverage using reporter 'text'|'html' statements: {Number:integer} // % of statements expected to be covered? };
Property check {Boolean:false}
Set this to true, if coverage testing should be done and fail, if limits that have been set are not reached.
Property dir {String:path}
Path to a directory, where coverage reports are written to. The directory is relative to the project directory (identified by package.json file).
Property perfile {Boolean:false}
Coverage thresholds must be reached by every instrumented source file.
Property reporter {Array<string>}
An array of strings, which lists all coverage reporters, that should be invoked. Check nyc and istanbul for coverage reporters.
Threshold properties:
branches
functions
lines
statements
For each of the above property, a percentage value [0 to 100] can be set, which is expected to be reached.
If testing uncovers a threshold breach, grunt-nyc-mocha will report the violation and stop the build process.
nyc
Property excludes {Array<string>}
An array of files and directories to exclude from instrumentation.
// nyc section const nyc = { excludes: [ "gruntfile.js", ".conf/**/*.js", "src/test/**/*.js" ] };
nyc
The example to the left excludes configuration files and the tests, because you would not want to collect code coverage metrics from such files.
Property exec {String}
Path to the nyc executable (scriptfile).
This value usually is evaluated by grunt-nyc-mocha and needs not to be set by a programmer.
// nyc section const nyc = { exec: <false|path> };
grunt-nyc-mocha
Setting this property is required only for situations in which some other nyc executable should be used, than the one in the projects node_modules folder
Property extensions {Array<string>}
A list of additional extensions that nyc should handle (instrument).
// nyc section const nyc = { extensions: [ "xjs" ] };
nyc
Instrument additional file types, like *.xjs in the example to the left.
Property includes {Array<string>}
An array of files and directories to include.
// nyc section const nyc = { includes: [ "src/scripts/**/*.js" ] };
nyc
Instrument files from additional directories, like some script directory in the example to the left.
Property requires {Array<string>}
An array of scripts to additionally require.
// nyc section const nyc = { requires: [ "grunt-nyc-mocha/scripts/sourcemapsupport" ] };
nyc
Require additonal scripts, before running instrumentation.
The example to the left loads nyc sourcemapsupport, to enable stacktraces point to original sourcefiles rather than instrumented code.
Property sourcemap {Object}
The sourcemap object bundles all properties which influence sourcemap handling.
// nyc section const sourcemap = { create: {Boolean}, // should nyc produce sourcemaps? use: {Boolean} // should nyc detect and handle sourcemaps? };
Property create {Boolean:false}
Set this to true, if nyc should create sourcemaps.
Property use {Boolean:false}
Set this to true, if nyc should detect and handle sourcemaps.
nyc
Usually the options to the left are not required for enabling sourcemap support with nyc.
Property temp {string}
Enables setting a directory where raw coverage data is written to.
// nyc section const nyc = { temp: ".raw_nyc" };
nyc
Write raw coverage data to directory “.raw_nyc”.
Property mocha {Object}
The mocha property is of type object and bundles all properties, which later will be passed on to the third-party-tool mocha.
// nyc section const mocha = { bail: {Boolean}, // abort ("bail") after first test failure color: {Boolean}, // force colored output exec: {String}, // path to node_modules/.../mocha script exit: {Boolean}, // force Mocha to quit after tests complete opts: {Array<string>}, // additional mocha options not coveredby plugin recursive: {Boolean}, // look for tests in subdirectories timeout: {Number:integer} // test timeout threshold (millis) };
grunt-nyc-mocha
Which parameters can be passed from grunt-nyc-mocha to mocha?
All!
The configuration section mocha contains a property
, which can be passed an array of strings. All strings in the array will be passed to mocha if the task is called.opts
Property bail {Boolean:false}
Abort with failure on first test failure.
// mocha section const mocha = { bail: false; };
mocha
Property color {Boolean:false}
Force colorized console output.
// mocha section const mocha = { color: true; };
mocha
Property exec {String}
Path to “node_modules/…/mocha”, in case some other installation should be used.
This property should remain untouched. Usually mocha can be located automatically.
// mocha section const mocha = { exec: "use/other/node_modules/bin/mocha"; };
mocha
Property exit {Boolean:false}
Force mocha to quit after tests completed.
There may be circumstances which keep processes running, until after the test suite is done. In these rare cases exit can be set to true, to force the ending of all processes as soon as all tests are run.
// mocha section const mocha = { exit: false; };
mocha
Property recursive {Boolean:false}
Look for tests in subdirectories.
This is not useful for grunt calls, if the src property already uses a gob (directory structure with wildcards).
// mocha section const mocha = { recursive: false; };
mocha
Property timeout {Number:integer:2000}
Set the test timeout threshold to “n” millis.
// mocha section const mocha = { timeout: 4000; };